Drag Files Here to Upload Orclick This Window to Open Your File Browser.
Editor's note: This post was updated on 24 March 2022 to include information on creating a drag-and-drop component using react-dropzone
and comparison it to the HTML Drag and Drop API.
In this tutorial, we'll walk through how to create a drag-and-drop component for uploading images using react-dropzone
. Our drag-and-drop component will include a regular paradigm click and select functionality.
We'll demonstrate the post-obit:
-
-
- Creating drag-and-drib functionality
-
-
- Listening for elevate and drop
- Detecting when a file is dropped on the drop zone
- Displaying the epitome proper noun and file blazon
- Ensuring images persist
- Validating dropped images
- Deleting duplicate images
- Removing unwanted images prior to upload
-
- Previewing images prior to upload
- Uploading images
-
react-dropzone
uses React hooks to create HTML5-compliant React components for handling the dragging and dropping of files. react-dropzone
provides the added functionality of restricting file types and also customizing the dropzone. With react-dropzone
, we no longer have to rely on the HTML Drag and Drop API. In lodge to showcase the simplicity of react-dropzone
, we'll also demonstrate the same tutorial using the HTML Drag and Drop API.
To follow along with the code for the react-dropzone version of this projection, go to GitHub.
The last issue volition look like this:
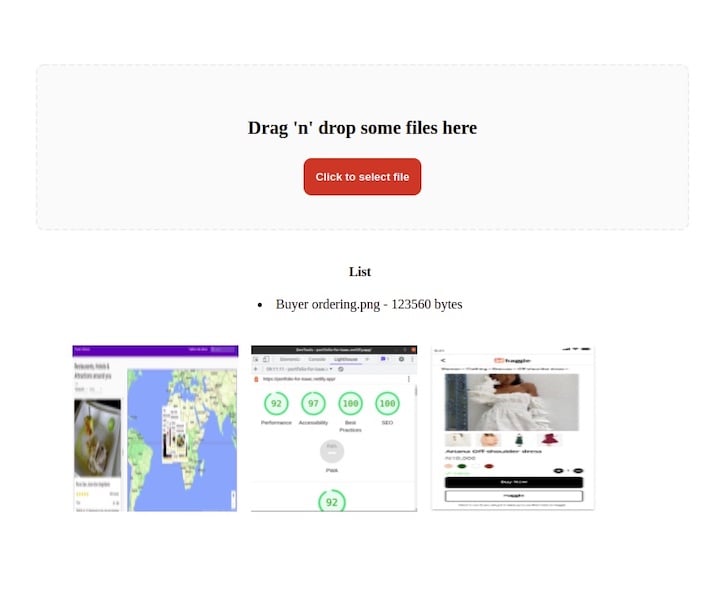
react-dropzone
.Getting started
This tutorial assumes that yous have Node.js
installed on your machine. Open your terminal, navigate to the directory where you desire to add your project and type the following:
npx create-react-app react-dropzone
Next, we'll install react-dropzone
into our application, equally follows:
// with npm npm install --save react-dropzone // with yarn yarn add react-dropzone
Create the drag-and-drib component with react-dropzone
react-dropzone
provides some prepare-made code snippets. To create a drag-and-drop component with react-dropzone
, all we demand to exercise is copy and paste the snippets in our App.js
file:
import React, {useEffect, useState} from 'react'; import {useDropzone} from 'react-dropzone'; part App(props) { const [files, setFiles] = useState([]); const {getRootProps, getInputProps} = useDropzone({ accept: 'image/*', onDrop: acceptedFiles => { setFiles(acceptedFiles.map(file => Object.assign(file, { preview: URL.createObjectURL(file) }))); } }); const thumbs = files.map(file => ( <div mode={thumb} key={file.name}> <div style={thumbInner}> <img src={file.preview} style={img} /> </div> </div> )); useEffect(() => { // Brand sure to revoke the data uris to avoid memory leaks files.forEach(file => URL.revokeObjectURL(file.preview)); }, [files]); return ( <section className="container"> <div {...getRootProps({className: 'dropzone'})}> <input {...getInputProps()} /> <p>Drag 'n' drop some files here, or click to select files</p> </div> <aside style={thumbsContainer}> {thumbs} </aside> </section> ); } export default App
The above code renders an image preview afterwards dragging and dropping.
Create drag-and-driblet and paradigm preview functionality
In the previous section, we demonstrated how like shooting fish in a barrel it is to create a drag-and-drop component with react-dropzone
. However, we did not address the issue of persisting images. When nosotros run npm start
to see our application in the browser, the application initially appears to run smoothly. Even so, nosotros see that if we drag a second image the starting time prototype does not persist on the page.
In social club to persist the dragged images, we will overhaul our previous code.
In our App.js
file, let's articulate everything out and then paste in the lawmaking below:
import React, { useCallback, useState } from 'react'; function App() { const [images, setImages] = useState([]); const onDrop = useCallback((acceptedFiles) => { acceptedFiles.map((file, alphabetize) => { const reader = new FileReader(); reader.onload = part (east) { setImages((prevState) => [ ...prevState, { id: index, src: e.target.upshot }, ]); }; reader.readAsDataURL(file); return file; }); }, []); return ( <div className="App"> </div> ); } export default App;
In the code higher up, we initialize an prototype land which nosotros set to an empty array, and then using the onDrop() callback
claw and we loop through our files if they are acceptable and if they are, we initialize a browser FileReader API and add the epitome to the state.
Next, nosotros'll create a DropBox
component and so pass the onDrop
which we will become from the App.js
file as a prop.
import { useDropzone } from 'react-dropzone'; import styled from 'styled-components'; const getColor = (props) => { if (props.isDragAccept) { return '#00e676'; } if (props.isDragReject) { return '#ff1744'; } if (props.isFocused) { return '#2196f3'; } return '#eeeeee'; }; const Container = styled.div` flex: 1; display: flex; flex-direction: column; marshal-items: centre; padding: 40px; edge-width: 2px; edge-radius: 10px; edge-color: ${(props) => getColor(props)}; edge-style: dashed; groundwork-color: #fafafa; color: black; font-weight: bold; font-size: 1.4rem; outline: none; transition: border 0.24s ease-in-out; `; function DropBox({ onDrop }) { const { getRootProps, getInputProps, acceptedFiles, open up, isDragAccept, isFocused, isDragReject, } = useDropzone({ take: 'image/*', onDrop, noClick: true, noKeyboard: true, }); const lists = acceptedFiles.map((listing) => ( <li key={list.path}> {list.path} - {list.size} bytes </li> )); return ( <> {' '} <section className="dropbox"> <Container className="dropbox" {...getRootProps({ isDragAccept, isFocused, isDragReject })} > <input {...getInputProps()} /> <p>Drag 'northward' drop some files here</p> <button type="push button" className="btn" onClick={open}> Click to select file </button> </Container> </department> <aside> <h4>List</h4> <p>{lists}</p> </aside> </> ); } export default DropBox;
In the DropBox component, we import useDropZone
from react-dropzone then we employ it equally a hook in which we destructured out *getRootProps*
, *open up*
, *getInputProps*
, *acceptedFiles*
props.
The getRootProps
is used to get the props that are required for drag-and-drop functionality and utilize it on any element.
The open up
prop is passed to the push to enable it to open the file directory to allow uploads.
The getInputProps
is likewise used to create the elevate-and-drop zone. Nevertheless, it must be practical to an input tag and information technology must have the spread operator to add the content returned from getInputProps
equally separate items to the input tag.
The acceptedFiles
props is used to cheque whether the files are accepted. We also mapped through it to render the file blazon and size in a list.
The noClick
and noKeyboard
set to true is to avoid opening the file manager by clicking on the DropBox
or by pressing the enter
and space
keys
We also installed styled components which nosotros will use in styling the DropBox
component.
Adjacent, create an Image.jsx
file for each image and paste in the code beneath:
import React from "react"; function Image({ epitome }) { return ( <div> <img alt='' src={prototype.src} /> </div> ); } export default Image;
Next, we create a ShowImage.jsx
file to show the image in a listing and also persist when we elevate and drib some other. Copy the following lawmaking into the ShowImage.jsx
file:
import Paradigm from './Image'; const ShowImage = ({ images }) => { const show = (paradigm) => { return <Epitome prototype={image} />; }; return <div className="container">{images.map(show)}</div>; }; export default ShowImage;
In the code above, we are importing the Paradigm
component and using it equally a wrapper to pass in the images
which nosotros volition be getting as props from the App.js
file
Next, we import the DropBox
and ShowImage
files into the App.js
file, laissez passer in the images into the ShowImage
component, and also pass onDrop
in to the DropBox
component.
import React, { useCallback, useState } from 'react'; import ShowImage from './ShowImage'; import DropBox from './DropBox'; function App() { // Country, browser FileReader and iterating return ( <div className="App"> <DropBox onDrop={onDrop} /> <ShowImage images={images} /> </div> ); } consign default App;
Now, permit's add some styling. We'll copy and paste the following styles below into our index.css
file:
torso { text-marshal: center; } .dropbox { text-align: center; padding: 20px; width: 90%; margin: machine; margin-top: 50px; } .container { display: flex; flex-wrap: wrap; width: 80%; margin: 20px motorcar; padding: 20px; } .container img { pinnacle: 200px; width: 200px; margin-right: 15px; } .btn { padding: 15px; background-color: #de1a1a; colour: white; font-weight: bold; border-radius: 10px; edge: none; cursor: pointer; } .btn:hover{ groundwork-color: #945c5c; }
Add prototype upload functionality
There are a lot of free image services, such as Cloudinary, but for this project, nosotros'll use imgbb. Create an account and then obtain an API key.
In the DropBox.js
file, we'll create a push button to handle the upload of our files to imgbb. Then, nosotros'll create an onClick method and pass in an updateFiles
function that will exist in charge of uploading the images when the button is clicked.
<button className="upload-btn" onClick={() => uploadFiles()}>Upload Images</button>
The uploadFiles
role:
const uploadFiles = () => { };
Adjacent, we'll create a land and set information technology to an empty array:
const [imageSent, setImageSent] = useState([]);
In the getInputProps()
inside the input tag, nosotros pass in an onChange
method pointing to a handleFile
part
<input {...getInputProps({ onChange: handleFile, })} />
The handleFile
function
const handleFile = (e) => { setImageSent(e.target.files[0]); };
Because it is an input tag for a file, we tin can access the event.target.files
and then phone call setImageSent
and update the country.
In order to make a request with the imgbb API, a cardinal and image properties are required. The key
is the API key we obtained before and the image is the file to exist uploaded.
To ready these properties, we'll utilize the FormData
interface. This tool provides a mode to easily construct key/value
pairs to correspond form fields and their values, which can then be easily sent.
Create a new FormData
within the uploadFiles
functon and suspend a central with the proper name image
and value imageSent
. Then, suspend another key named key
. The value should exist your API key.
We'll use Axios
to handle our POST
asking, but this can too be done with fetch
:
const uploadFiles = () => { const formData = new FormData(); console.log(imageSent); formData.append('image', imageSent); formData.append('key', 'Your Api central goes hither'); Axios.post('https://api.imgbb.com/ane/upload', formData).then((response) => { console.log(response); }); };
That's information technology! We've created our drag-and-drop paradigm uploader with react-dropzone
!
As a basis for comparison, allow's accept a wait at the aforementioned tutorial using the HTML Drag and Drib API.
Create the drag-and-drop component with Dropzone
To create a Dropzone component, we would create a folder chosen Dropzone
inside the src
folder and add two files: Dropzone.js
and Dropzone.css
.
Then, we'd add an arrow function chosen Dropzone
within the Dropzone.js
file and set the consign function
as default. Nosotros'd set up the parent element to empty tags.
import React from 'react'; const Dropzone = () => { render ( <> </> ) } consign default Dropzone;
Adjacent, nosotros'd import the Dropzone
component into the App.js
file:
import Dropzone from "./Dropzone/Dropzone";
We'd add the component as a kid of the div
element with class name content
:
return ( <div> <p className="title">React Drag and Drop Paradigm Upload</p> <div className="content"> <Dropzone /> </div> </div> );
Now back to the Dropzone
component. We'd add together a div
element with grade name container
. then, inside the container component, we'd add a div
with class proper name drop-container
.
<div className="container"> <div className="drib-container"> </div> </div>
Next, we'd add this CSS style into the Dropzone.css
and import the file within the component:
.container { transform: translateY(-100%); } .container p { color: reddish; text-align: centre; } .drop-container { brandish: flex; align-items: centre; justify-content: center; margin: 0; width: 800px; height: 200px; border: 4px dashed #4aa1f3; }
Then, inside the div
with class name driblet-container
, nosotros'd add together the post-obit elements:
<div className="driblet-message"> <div className="upload-icon"></div> Drag & Drib files here or click to upload </div> .upload-icon { width: 50px; height: 50px; background: url(../images/upload.png) no-repeat center center; background-size: 100%; text-align: center; margin: 0 car; padding-top: 30px; } .driblet-bulletin { text-align: center; color: #4aa1f3; font-family unit: Arial; font-size: 20px; }
Create drag-and-drop functionality with the HTML elevate-and-drib API
HTML Drag-and-Drop uses the DOM issue model and elevate events inherited from mouse events.
A drag functioning begins when a user selects an particular from the Bone, drags the detail to a droppable element, and so releases the dragged particular. During elevate operations, several events are fired. Some events might fire multiple times.
The elevate-and-drop API defines eight events: four events for the draggable chemical element and iv events for the droppable chemical element. For this illustrative instance, we'd simply need the four events for the droppable chemical element. Each drag event type has an associated event handler. The events are:
dragenter — A dragged particular enters a valid drib target (ondragenter) dragleave — A dragged item leaves a valid drop target (ondragleave) dragover — A dragged item is dragged over a valid drop target every few hundred milliseconds (ondragover) drop — an item is dropped on a valid drib target (ondrop)
So far, nosotros've specified the drop region for our files, only there's no valid region in which to drop them. When a file is dragged into a browser from the OS, the browser will attempt to open and brandish it by default. If we wanted to allow a drib, nosotros'd need to forbid the default handling of the outcome handlers.
On the div
chemical element with class proper name driblet-container
, nosotros'd add four event methods.
onDragOver={dragOver} onDragEnter={dragEnter} onDragLeave={dragLeave} onDrop={fileDrop}
In the beneath lawmaking, we'd have the event handler methods listed on the left and the methods to handle event handlers listed on the right. The div
element would expect as follows:
<div className="drop-container" onDragOver={dragOver} onDragEnter={dragEnter} onDragLeave={dragLeave} onDrop={fileDrop} > ... </div>
Nosotros'd define the methods for handling the events:
const dragOver = (east) => { e.preventDefault(); } const dragEnter = (eastward) => { e.preventDefault(); } const dragLeave = (east) => { e.preventDefault(); } const fileDrop = (e) => { e.preventDefault(); const files = eastward.dataTransfer.files; console.log(files); }
The e.dataTransfer
is a Data Transfer
object that holds the information that is being dragged during a drag-and-drop operation. It may hold one or more than information items. e.dataTransfer.files
contains the dragged local files as a FileList
.
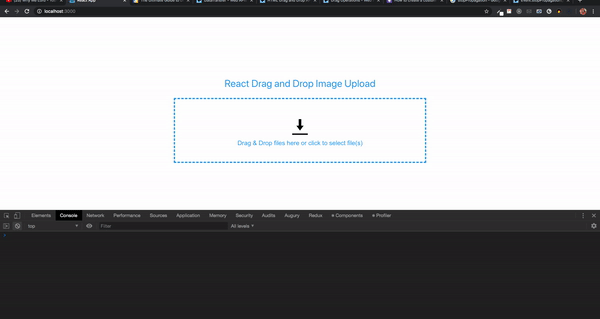
Next, nosotros'd need to handle the files from the FileList
by validating the file type, checking the file size, and displaying the file name.
Validate and bank check file sizes
In this example, we're simply calculation image files. That's because the storage platform that we'll use later to store the uploaded files only allows mostly image files. Permit'south expect at validating the files.
Get-go, we'd create a method chosen handleFiles
and add it to fileDrop
with files
as a parameter.
const handleFiles = (files) => { }
Next, we'd add to fileDrop
const fileDrop = (east) => { e.preventDefault(); const files = e.dataTransfer.files; if (files.length) { handleFiles(files); } }
We'd create a new method called validateFile
with a parameter called file
. The method will return a Boolean value.
const validateFile = (file) => { const validTypes = ['image/jpeg', 'paradigm/jpg', 'image/png', 'image/gif', 'image/x-icon']; if (validTypes.indexOf(file.type) === -1) { return faux; } return true; }
Here nosotros have an array with the file types. You tin can add together or remove any blazon. The file
parameter from the FileList
contains a type property. When using JavaScript'due south indexOf
method, if the type is not found in the assortment, it returns -1
; otherwise, information technology returns the index of the value in the array. It returns imitation
if the type is non institute and true
if it is found.
At present, nosotros'd use the validateFile
method inside handleFiles
. The FileList
is an array, and we'd loop through the array:
for(permit i = 0; i < files.length; i++) { if (validateFile(files[i])) { // add to an array so we can display the name of file } else { // add a new property called invalid // add to the aforementioned array so we can display the name of the file // set fault message } }
Then, nosotros'd import the useState
hook from react
:
import React, { useState } from 'react';
Next, we'd add two state variables inside the Dropzone
function:
const [selectedFiles, setSelectedFiles] = useState([]); const [errorMessage, setErrorMessage] = useState(''); selectedFiles has a type of assortment while errorMessage has a type of string. // add a new property called invalid files\[i\]['invalid'] = true;
Now, we'd update the selectedFiles
assortment with the new object containing an invalid property:
// add to the aforementioned array and so we can display the name of the file setSelectedFiles(prevArray => [...prevArray, files[i]]);
Hither we'd use a callback inside the setSelectedFiles
method in lodge to become a quick update to the array:
We'd add the error bulletin.
// set error message setErrorMessage('File type not permitted');
Before we tin try this out, we'd need to add a div
to display the files inside the selectedFiles
array. Next, we'd add the post-obit after the div
with grade name driblet-container
.
<div className="file-display-container"> <div className="file-status-bar"> <div> <div className="file-type-logo"></div> <div className="file-blazon">png</div> <span className="file-name">test-file.png</span> <bridge className="file-size">(twenty.5 KB)</span> {<bridge className='file-fault-message'>(File type non permitted)</span>} </div> <div className="file-remove">X</div> </div> </div>
Now, we'd add these styles to the CSS file for Dropzone
:
.file-brandish-container { position: fixed; width: 805px; } .file-status-bar{ width: 100%; vertical-align:top; margin-summit: 10px; margin-bottom: 20px; position: relative; line-superlative: 50px; height: 50px; } .file-condition-bar > div { overflow: subconscious; } .file-type { display: inline-block!of import; position: absolute; font-size: 12px; font-weight: 700; line-height: 13px; margin-top: 25px; padding: 0 4px; edge-radius: 2px; box-shadow: 1px 1px 2px #abc; color: #fff; background: #0080c8; text-transform: uppercase; } .file-name { display: inline-block; vertical-align:acme; margin-left: 50px; color: #4aa1f3; } .file-fault { display: inline-block; vertical-align: top; margin-left: 50px; color: #9aa9bb; } .file-fault-message { color: cerise; } .file-type-logo { width: 50px; summit: 50px; background: url(../images/generic.png) no-echo center center; background-size: 100%; position: absolute; } .file-size { display:inline-cake; vertical-align:acme; color:#30693D; margin-left:10px; margin-right:5px; margin-left: 10px; color: #444242; font-weight: 700; font-size: 14px; } .file-remove { position: absolute; height: 20px; correct: 10px; line-meridian: 15px; cursor: pointer; color: reddish; margin-right: -10px; }
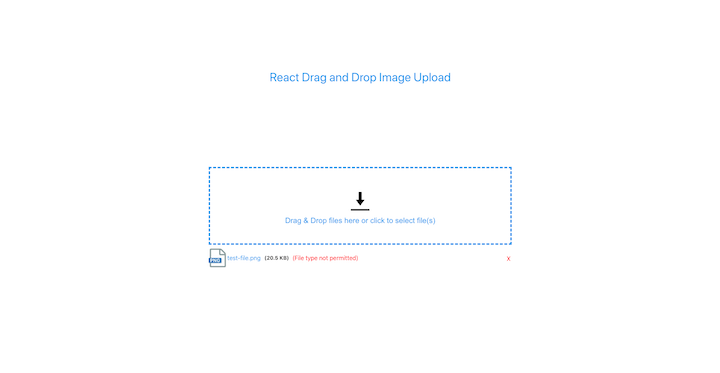
We can run into how the files will be displayed. The file type would be extracted and displayed, as Ould the file name and size. The error message would only be displayed for invalid files.
Now that we have placeholders for the files that will exist displayed on the page, we could utilise the selectedFiles
array within the template.
Outset, we'd create a method called fileSize
. This would accept in a size parameter. The file object from FileList
contains a size holding.
const fileSize = (size) => { if (size === 0) return '0 Bytes'; const k = 1024; const sizes = ['Bytes', 'KB', 'MB', 'GB', 'TB']; const i = Math.flooring(Math.log(size) / Math.log(k)); return parseFloat((size / Math.pow(k, i)).toFixed(2)) + ' ' + sizes[i]; }
In the above code, nosotros return the string 0 Bytes
if the file size is zero. 1kB is equivalent to 1024 bytes. We tin calculate the natural log of the size past dividing by the natural log of bytes value. Math.flooring
returns an integer. The render value of the function is the size divided past the value of yard
to the ability of i
with the sizes value appended.
Next, we'd add a method for getting the file blazon from the file name:
const fileType = (fileName) => { return fileName.substring(fileName.lastIndexOf('.') + 1, fileName.length) || fileName; }
The substring()
method extracts the characters from a string between two specified indices and returns the new substring. The substring()
method returns the characters after the .
in the file name.
<div className="file-display-container"> { selectedFiles.map((information, i) => <div className="file-status-bar" key={i}> <div> <div className="file-type-logo"></div> <div className="file-type">{fileType(data.proper name)}</div> <span className={`file-name ${information.invalid ? 'file-mistake' : ''}`}>{data.proper name}</span> <bridge className="file-size">({fileSize(information.size)})</span> {data.invalid && <bridge className='file-error-message'>({errorMessage})</span>} </div> <div className="file-remove">X</div> </div> ) } </div>
Now, nosotros'd loop through the selectedFiles
array. We'll use the fileType
method past passing the file name.
<span className={`file-proper noun ${data.invalid ? 'file-error' : ''}`}>{information.proper noun}</span>
Adjacent, nosotros'd check whether the object contains the invalid
property added, which would indicate an invalid file. We'd add the form name file-error
.
<bridge className="file-size">({fileSize(information.size)})</span> {data.invalid && <span className='file-mistake-message'>({errorMessage})</bridge>}
Nosotros'd display the file size by using the fileSize
method. The error message would be displayed side by side to it if it is an invalid file.
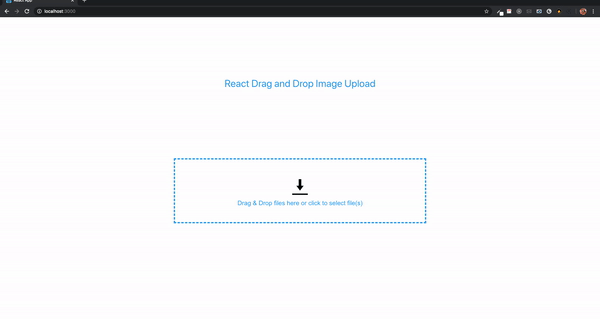
Adding valid files is simple. Inside the if
role of the handleFiles
method, we'd add the post-obit:
setSelectedFiles(prevArray => [...prevArray, files[i]]);
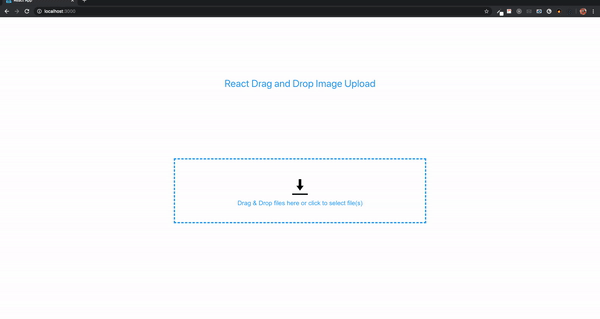
We could even drag and drop multiple files at the same time.
Delete duplicate valid files
One drawback of the selectedFiles
array is that a detail file can exist added multiple times. We don't want this behavior.
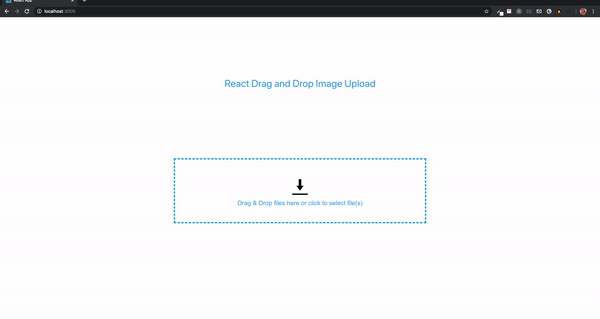
To remove duplicates from the selectedFiles
array, we'd add together a new useState
variable inside the component.
const [validFiles, setValidFiles] = useState([]);
Next, we'd import the useEffect
claw:
import React, { ..., useEffect } from 'react';
Now, we'd utilise the JavaScript reduce
, discover
, and concat
methods to remove duplicates and add the individual values into the new array validFiles
:
useEffect(() => { permit filteredArray = selectedFiles.reduce((file, electric current) => { const x = file.find(particular => item.proper name === current.name); if (!x) { return file.concat([electric current]); } else { return file; } }, []); setValidFiles([...filteredArray]); }, [selectedFiles]);
The outcome of filteredArray
would exist used to update the validFiles
array. Next, we'd replace the selectedFiles
in the HTML map to validFiles
:
<div className="file-display-container"> { validFiles.map((data, i) => ... ) } </div>
Now, we'd check to ensure that a particular file can be added only once.
Remove unwanted files prior to upload
Before uploading the images, users should take the option of removing images from the list. We already accept a button for removing an detail from the list.
To create this functionality, we'd start add a method chosen removeFile
with the file name as a parameter on the div
element with course name: file-remove
.
<div className="file-remove" onClick={() => removeFile(data.proper noun)}>X</div>
Next, we'd need to remove the selected file from the validFiles
and selectedFiles
arrays. Nosotros'd use the JavaScript findIndex
method to find the index of the file. Then, we'd utilise the splice
method to remove the item from the arrays and update information technology with the setValidFiles
and setSelectedFiles
methods.
const removeFile = (name) => { // find the alphabetize of the item // remove the detail from array const validFileIndex = validFiles.findIndex(e => e.name === name); validFiles.splice(validFileIndex, ane); // update validFiles array setValidFiles([...validFiles]); const selectedFileIndex = selectedFiles.findIndex(e => e.name === name); selectedFiles.splice(selectedFileIndex, 1); // update selectedFiles array setSelectedFiles([...selectedFiles]); }
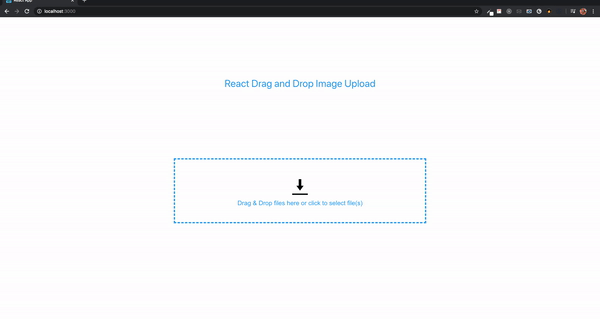
Preview images prior to upload
Nosotros could add a simple modal to preview an paradigm before all images are uploaded. The preview would only be for valid files. We could remove invalid files by clicking on their file proper noun. First, nosotros'd add the modal div
s after the div
with class name container
.
<div className="modal"> <div className="overlay"></div> <span className="close">X</bridge> <div className="modal-image"></div> </div>
The div
element with class name modal-paradigm
will brandish the paradigm. Adjacent, we'd add the CSS styles. By default, the modal display is gear up to not
due east. It will but be displayed when an image name is clicked.
.modal{ z-index: 999; display: none; overflow: subconscious; } .modal .overlay{ width: 100%; elevation: 100vh; groundwork: rgba(0,0,0,.66); position: absolute; meridian: 0; left: 0; } .modal .modal-epitome{ position: accented; top: fifty%; left: 50%; transform: interpret(-fifty%,-fifty%); overflow: hidden; object-fit: embrace; width: 100%; height: 300px; background-size: contain; groundwork-repeat: no-repeat; groundwork-position: center; } .shut { position: absolute; summit: 15px; right: 35px; color: #f1f1f1; font-size: 40px; font-weight: bold; transition: 0.3s; }
Next, we'd add the beneath code on the div
inside the element with class proper name: file-status-bar
.
onClick={!data.invalid ? () => openImageModal(information) : () => removeFile(data.name)} <div className="file-display-container"> { validFiles.map((data, i) => <div className="file-status-bar" key={i}> <div onClick={!data.invalid ? () => openImageModal(data) : () => removeFile(data.name)}> ... </div> </div> ) } </div>
The openImageModal
would display valid files while invalid files are removed when clicked on. Next, nosotros'd add the method openImageModal
:
const openImageModal = (file) => { }
Now, we'd import the useRef
Hook from React. The ref
will allow us to display the modal, show the image, and close the modal.
import React, { ..., useRef } from 'react';
Next, we'd add the following refs
variables:
const modalImageRef = useRef(); const modalRef = useRef();
On the modal elements, nosotros'd add the respective ref
s.
<div className="modal" ref={modalRef}> <div className="overlay"></div> <span className="shut">X</bridge> <div className="modal-image" ref={modalImageRef}></div> </div> modalRef is used to display and hide the modal element and its contents modalImageRef displays the image
Nosotros'd need to read the content of the file passed every bit a parameter into the openImageModal
. At present, we'll add the FileReader
constructor.
const reader = new FileReader();
The [FileReader](https://developer.mozilla.org/en-US/docs/Web/API/FileReader)
object enables web applications to asynchronously read the contents of files (or raw data buffers) stored on the user's reckoner using File
or Hulk
objects to specify the file or information to read.
Adjacent, nosotros'd gear up the display of the modal to block
using the modalRef
.
const reader = new FileReader(); modalRef.current.way.brandish = "cake";
We'd need a mode to read the content of the file using readAsDataURL
and add an consequence handler to be triggered one time the reading performance is complete.
const reader = new FileReader(); modalRef.electric current.style.display = "block"; reader.readAsDataURL(file); reader.onload = office(east) { modalImageRef.current.way.backgroundImage = `url(${e.target.result})`; }
The e.target.result
aspect contains a data:
URL representing the file's information. We'd gear up that as the background image of the div
with ref modalImageRef
.
In the CSS mode for modal-image
, we've already set the width, superlative, and some background properties.
Next, nosotros'd add an onClick
method on the span element with class name shut
.
<span className="close" onClick={(() => closeModal())}>10</span>
We'd create a method closeModal
. When this method is called, the modal display is ready to none and the epitome groundwork is set to none so as to reset.
const closeModal = () => { modalRef.current.fashion.display = "none"; modalImageRef.electric current.way.backgroundImage = 'none'; }
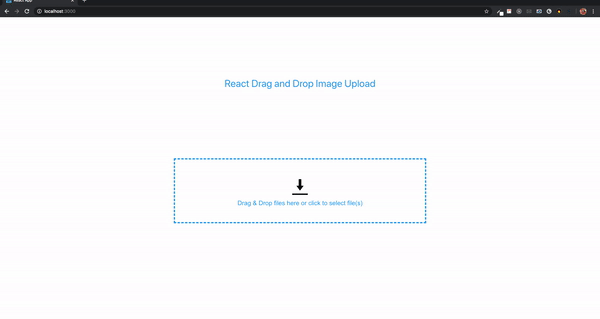
Upload images
In order to provide the ability to upload images, we'd need to create a display upload button and build the functionality to allow users to click to select particular files.
Create a display upload push
Showtime, we'd add together an upload push button. The button will only be displayed if all files are valid. If there is at least one invalid file, a bulletin would exist displayed instead.
First, we'd add together a push with grade proper noun file-upload-btn
as the first chemical element inside the container div
.
<button className="file-upload-btn">Upload Files</push> .file-upload-btn { color: white; text-transform: uppercase; outline: none; background-colour: #4aa1f3; font-weight: bold; padding: 8px 15px; margin-bottom: 5px; }
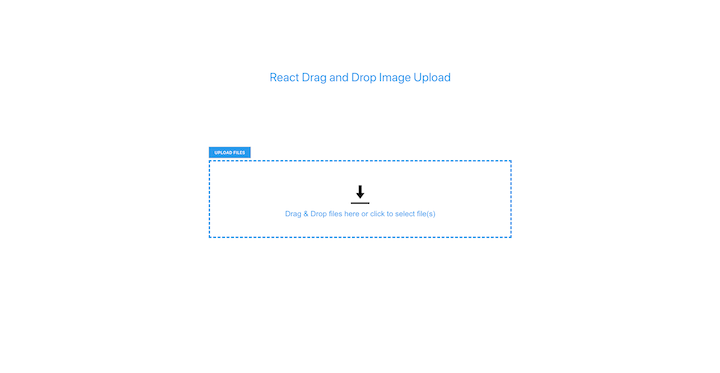
To hide and brandish the upload button, nosotros'd add a new useState
variable to concord all invalid files. If the array length is zero, the push button would be displayed; otherwise, the push button would exist hidden.
const [unsupportedFiles, setUnsupportedFiles] = useState([]);
We'd use the setUnsupportedFiles
the same fashion we used setSelectedFiles
. Showtime, we'd add the following within the else
portion of the handleFiles
and removeFile
methods:
const handleFiles = (files) => { for(let i = 0; i < files.length; i++) { if (validateFile(files[i])) { ... } else { ... ... ... setUnsupportedFiles(prevArray => [...prevArray, files[i]]); } } }
With the below code, we'd specify that each invalid file dropped by the user would be added to the assortment.
const removeFile = (name) => { // find the alphabetize of the item // remove the detail from assortment ... ... const unsupportedFileIndex = unsupportedFiles.findIndex(e => east.proper name === proper name); if (unsupportedFileIndex !== -1) { unsupportedFiles.splice(unsupportedFileIndex, ane); // update unsupportedFiles assortment setUnsupportedFiles([...unsupportedFiles]); } }
If the index of the element is institute, the item would exist spliced and unsupportedFiles
would exist updated.
Now, we'd supervene upon the upload push button with these ii lines:
{unsupportedFiles.length === 0 && validFiles.length ? <button className="file-upload-btn" onClick={() => uploadFiles()}>Upload Files</button> : ''} {unsupportedFiles.length ? <p>Please remove all unsupported files.</p> : ''}
-
-
- The showtime line checks whether the
unsupportedFiles
length is nothing andvalidFiles
array has at least one value. if these weather are met, the push button is displayed; otherwise, the push would exist hidden. - The 2nd line displays the bulletin if at that place is at to the lowest degree one invalid file.
Display upload button and error message.
- The showtime line checks whether the
-
Build click-to-select functionality
Before we add the upload functionality, nosotros'd need to provide users with the ability to click to select images. A hidden input field is added with type file
, an onChange
event method, and a ref
property. An onClick
method would exist added to the drop-container
so that when whatsoever function of the container is clicked, information technology would trigger the hidden input field by using its ref
.
First, we'd add an onClick
to driblet-container
:
onClick={fileInputClicked}
Adjacent, we'd add together an input field later on the drop-message
element:
<input ref={fileInputRef} className="file-input" blazon="file" multiple onChange={filesSelected} /> const fileInputRef = useRef(); .file-input { brandish: none; }
Now, nosotros'd add together a method called fileInputClicked
with fileInputRef.electric current.clicked
:
const fileInputClicked = () => { fileInputRef.current.click(); }
Side by side, we'd add together some other method for the filesSelected
. The selected files can exist obtained from fileInputRef.current.files
. We just need to pass it into the handleFiles
method.
const filesSelected = () => { if (fileInputRef.current.files.length) { handleFiles(fileInputRef.current.files); } }
With these methods, we'd be able to select multiple files.
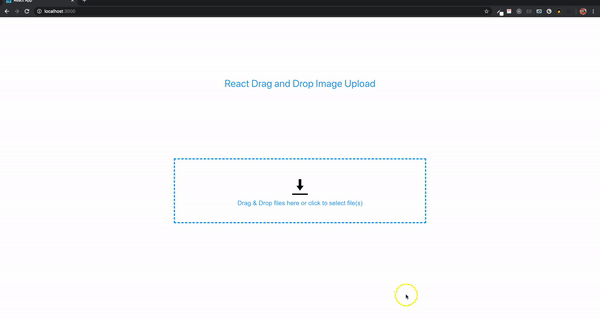
Add upload functionality
For the purpose of this demo, nosotros'd use a costless service called imgbb to add together the upload functionality to our Dropzone component. Beginning, nosotros'd create an account so obtain an API key from https://api.imgbb.com/.
Now, nosotros'd add a method called uploadFiles
to the upload button:
<button className="file-upload-btn" onClick={() => uploadFiles()}>Upload Files</button>
Adjacent, nosotros'd add the uploadFiles
method:
const uploadFiles = () => { }
A modal with a progress bar would be displayed when the upload button is clicked. Next, we'd add these upload modal elements:
<div className="upload-modal" ref={uploadModalRef}> <div className="overlay"></div> <div className="close" onClick={(() => closeUploadModal())}>10</div> <div className="progress-container"> <span ref={uploadRef}></span> <div className="progress"> <div className="progress-bar" ref={progressRef}></div> </div> </div> </div> .upload-modal { z-index: 999; display: none; overflow: hidden; } .upload-modal .overlay{ width: 100%; height: 100vh; groundwork: rgba(0,0,0,.66); position: absolute; pinnacle: 0; left: 0; } .progress-container { background: white; width: 500px; height: 300px; position: absolute; peak: l%; left: fifty%; transform: interpret(-50%,-50%); overflow: hidden; } .progress-container span { display: flex; justify-content: center; padding-top: 20px; font-size: 20px; } .progress { width: 90%; position: absolute; top: l%; left: l%; transform: interpret(-fifty%,-50%); background-color: #efefef; height: 20px; border-radius: 5px; } .progress-bar { position: absolute; background-color: #4aa1f3; height: 20px; edge-radius: 5px; text-align: center; colour: white; font-weight: bold; } .mistake { colour: cherry; }
The modal has elements with ref
south. Nosotros'd add the ref
variables besides as the closeUploadModal
method:
const uploadModalRef = useRef(); const uploadRef = useRef(); const progressRef = useRef(); uploadModalRef displays and hides the upload modal uploadRef shows messages progressRef updates the progress bar
Inside the closeUploadModal
method, set the display of uploadModalRef
to none.
const closeUploadModal = () => { uploadModalRef.electric current.way.display = 'none'; }
In the uploadFiles
method, we'd offset set up the brandish of uploadModalRef
to cake
. As well, we'd add the string File(s) Uploading...
to uploadRef
innerHTML
.
const uploadFiles = () => { uploadModalRef.current.mode.display = 'block'; uploadRef.electric current.innerHTML = 'File(s) Uploading...'; }
Since we already have the valid files within the validFiles
array, all we need to exercise is loop through the array, fix the right backdrop using FormData
, and then brand the request.
const uploadFiles = () => { uploadModalRef.electric current.style.display = 'block'; uploadRef.current.innerHTML = 'File(s) Uploading...'; for (permit i = 0; i < validFiles.length; i++) { } }
Co-ordinate to the imgbb API documentation, a fundamental and epitome backdrop are required in club to make the asking. The fundamental
is the API fundamental we obtained and the paradigm is the file to exist uploaded. Nosotros could catechumen to a binary file, base64 data, or a URL for an image.
To ready these properties, nosotros'd use the FormData
interface. This tool provides a fashion to easily construct fundamental/value
pairs to stand for form fields and their values, which can then be sent.
First, we'd create a new FormData
inside the for
loop and suspend a key with the proper noun image
and value validFiles[i]
. Then, we'd append some other primal named key
. The value would be our API key.
const uploadFiles = () => { uploadModalRef.current.style.display = 'cake'; uploadRef.current.innerHTML = 'File(s) Uploading...'; for (let i = 0; i < validFiles.length; i++) { const formData = new FormData(); formData.append('image', validFiles[i]); formData.append('cardinal', 'add together your API key here'); } }
To make the request, nosotros'd utilise Axios considering it has a method nosotros could use to become the upload progress. From this, the progress bar value could be calculated and displayed.
We'd run the following installation command:
npm install axios
Once installed, we'd import inside the Dropzone
component:
import axios from 'axios';
A post
request would be required to upload the file(s) to imgbb
:
const uploadFiles = () => { uploadModalRef.electric current.style.display = 'block'; uploadRef.electric current.innerHTML = 'File(south) Uploading...'; for (let i = 0; i < validFiles.length; i++) { const formData = new FormData(); formData.append('image', validFiles[i]); formData.append('key', 'add together your API key here'); axios.post('https://api.imgbb.com/1/upload', formData, {}) .catch(() => { // If error, display a bulletin on the upload modal uploadRef.current.innerHTML = `<span class="error">Error Uploading File(south)</span>`; // gear up progress bar groundwork color to red progressRef.current.style.backgroundColor = 'ruddy'; }); } }
Axios postal service
takes three parameters: the URL, data, and final object is where the outcome for the upload progress is calculated. Y'all tin learn more well-nigh the Axios asking configuration on GitHub. We're interested in the onUploadProgress
method, which facilitates the treatment of progress events for uploads.
Conclusion
In this guide, we walked through how to create a elevate-and-drop image uploader with react-dropzone
. We demonstrated the simplicity of this method compared to using the HTML Drag and Drib API. By reviewing the react-dropzone
official documentation and making a few tweaks to our code, nosotros can extend our application to accept other file types similar PDF, Zip, etc., depending on the storage service.
sharwooddosever76.blogspot.com
Source: https://blog.logrocket.com/create-drag-and-drop-component-react-dropzone/
0 Response to "Drag Files Here to Upload Orclick This Window to Open Your File Browser."
Post a Comment